Dependency injection (DI) has always been a key component in developing scalable and testable Android applications. While Dagger has been a popular choice for DI, Hilt—a DI framework built on top of Dagger—offers a simplified, powerful approach, especially for Android development. In 2024, Hilt has become the go-to framework for DI, streamlining app architecture and improving code maintainability. Here’s why you should consider implementing Hilt in every app you build this year.
What is Hilt?
Hilt is a dependency injection framework specifically tailored for Android. Built on Dagger, it simplifies DI by reducing the amount of boilerplate code required and providing predefined components and annotations suited for Android applications. Hilt integrates seamlessly with the Android framework and includes lifecycle management for dependencies, which makes it a convenient, optimized choice for developers.
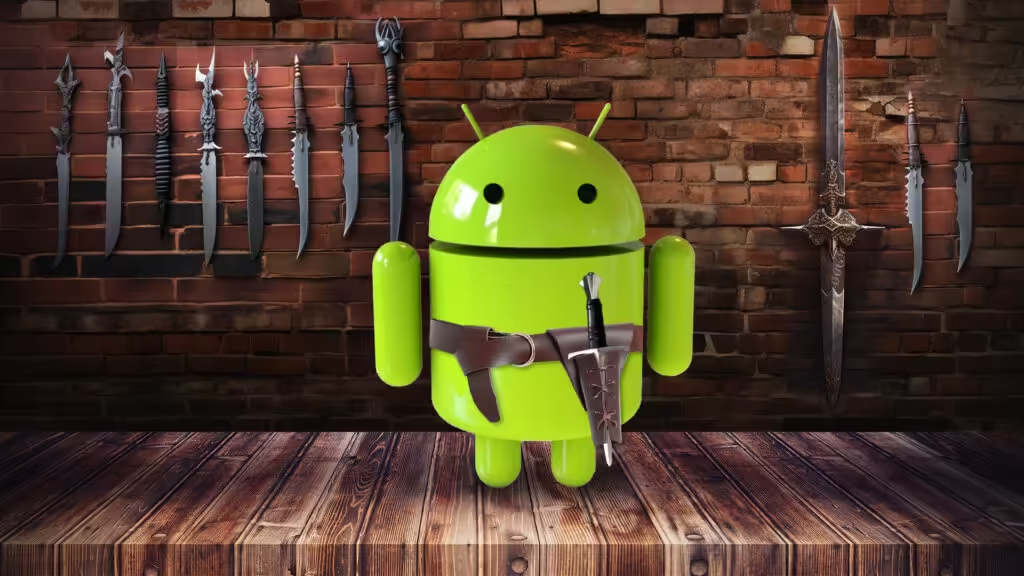
Why Hilt Matters for Android Development
1. Reduces Boilerplate Code
Using Dagger traditionally required a lot of setup with components, modules, and scopes. Hilt significantly simplifies this by providing built-in Android-specific components like @ApplicationScoped
and @ActivityScoped
, eliminating the need for custom component definitions. This reduction in boilerplate code allows developers to focus on app logic rather than dependency management.
Example: To inject a dependency in an Android activity, you only need to use the @AndroidEntryPoint
annotation on the activity, making it straightforward and intuitive.
@UninstallModules(NetworkModule::class)
@HiltAndroidTest
class UserRepositoryTest {
@Inject lateinit var fakeRepository: FakeUserRepository
}
2. Improves Testing Capabilities
Hilt is designed to make dependency injection straightforward, but it also excels at making dependencies testable. By utilizing Hilt’s @TestInstallIn
and @UninstallModules
annotations, you can easily swap out production implementations with test doubles.
For example, you can replace real network services with mock implementations during tests, providing better control over test cases and making unit and integration testing more reliable.
Example:
@UninstallModules(NetworkModule::class)
@HiltAndroidTest
class UserRepositoryTest {
@Inject lateinit var fakeRepository: FakeUserRepository
}
3. Optimized for Android’s Architecture
Hilt integrates directly with Android’s architecture components, including ViewModel, Navigation, and WorkManager. This integration is invaluable in 2024 as Android apps become more complex and architecture-focused. With Hilt, dependencies are scoped correctly to each Android class, like activities, fragments, and services. This eliminates memory leaks and makes the lifecycle management of dependencies more efficient.
4. Increases Code Readability and Maintainability
By using Hilt, developers can improve the readability of their code. With clear annotations like @Inject
and predefined scopes, Hilt makes dependency injection understandable at a glance. This approach is especially beneficial in large teams where multiple developers contribute to the codebase, making it easier for everyone to understand dependency flows without additional setup or documentation.
5. Enhances Performance
One of the main goals of DI frameworks is to improve app performance. Hilt is built on Dagger, which is highly optimized for performance. By utilizing compile-time dependency injection, Hilt avoids the overhead associated with reflection, resulting in faster and more efficient dependency management. This approach leads to smoother and faster Android applications.
6. Easier Migration for Existing Dagger Users
If you’re already using Dagger, Hilt makes the transition seamless by building on top of Dagger’s framework. You can start with a gradual migration, incorporating Hilt components and features one by one without a complete overhaul. This gradual integration ensures you can maintain productivity while adopting Hilt.
Key Features in Hilt for 2024
Several Hilt updates have made it an indispensable tool for Android developers in 2024:
- ViewModel Injection: Direct injection into ViewModels using
@ViewModelInject
is supported, making it easy to manage dependencies without requiring ViewModel factories. - Simplified WorkManager Integration: Hilt now allows WorkManager dependencies to be injected directly, reducing the setup process for background tasks.
- Custom Entry Points: Advanced Hilt users can leverage custom entry points to inject dependencies into classes outside of the Android lifecycle, such as helper classes and third-party libraries.
- Annotation Processing Optimizations: Hilt’s annotation processing has been optimized, speeding up build times and improving performance across projects of all sizes.
Practical Example: Setting Up Hilt in Your Android App
Setting up Hilt is easy. Start by adding the required dependencies to your build.gradle
file:
implementation 'com.google.dagger:hilt-android:2.44'
kapt 'com.google.dagger:hilt-android-compiler:2.44'
Then, annotate your application class with @HiltAndroidApp
to set up Hilt for the entire app:
@HiltAndroidApp
class MyApplication : Application()
Next, annotate your Android components (activities, fragments) with @AndroidEntryPoint
, and use @Inject
to inject dependencies as needed.
@AndroidEntryPoint
class HomeFragment : Fragment() {
@Inject lateinit var userRepository: UserRepository
}
With this setup, Hilt will automatically handle the lifecycle of dependencies, creating a cleaner and more efficient codebase.
Internal and External Links
For further reading on dependency injection and Hilt, check out these resources:
External Resources:
Conclusion
As we move into 2024, Hilt has proven itself to be the best choice for dependency injection in Android apps. It offers simplicity, performance, and optimized support for Android’s architecture components. For Android developers looking to improve the maintainability, testability, and performance of their applications, Hilt is a must-have tool in your development toolkit. By incorporating Hilt, you’re setting up your app for success, both now and in the years to come.