With the release of Swift 6.0 and iOS 18, Apple continues to push the boundaries of app performance and developer productivity. One of the most important advancements is the enhanced async/await functionality, which allows for cleaner and more efficient handling of asynchronous operations. Since its introduction in Swift 5.5, async/await has evolved into a core feature for managing concurrency in modern iOS development.
In this post, we’ll explore how to master async/await in Swift 6.0 for iOS 18 apps. You’ll learn how to streamline your code, improve performance, and create a more responsive user experience by leveraging the latest concurrency features.
Why Async/Await Matters for iOS 18 Development
Concurrency has always been a challenge in mobile app development. From network requests to file handling and UI updates, many tasks in iOS apps require asynchronous code execution. Before the introduction of async/await, developers relied on complex closures and completion handlers, which often resulted in callback hell and harder-to-read code.
Async/await changes the game by providing a simple and readable syntax that allows you to write asynchronous code just like synchronous code. With Swift 6.0, the async/await system has been further refined to:
• Improve performance by minimizing thread-blocking.
• Reduce code complexity, making it easier to maintain large codebases.
• Enhance developer productivity, enabling faster app iteration.
For iOS 18 apps, adopting async/await will help create snappier, more responsive experiences for users while simplifying your code logic.
Getting Started with Async/Await in Swift 6.0
At its core, async and await are used to handle asynchronous tasks in a more intuitive manner. In Swift 6.0, the syntax remains consistent, but there are improvements in how the language handles tasks, task cancellation, and structured concurrency.
Here’s a simple example to illustrate the basics of async/await:
func fetchData() async throws -> Data {
let url = URL(string: "https://example.com/api/data")!
let (data, _) = try await URLSession.shared.data(from: url)
return data
}
Task {
do {
let result = try await fetchData()
print("Data received: \(result)")
} catch {
print("Error fetching data: \(error)")
}
}
In this example:
• The fetchData() function uses async and await to perform a network call.
• Task is used to create a new asynchronous context for calling the function.
Key Concepts in Swift 6.0 Async/Await
1. Structured Concurrency
With Swift 6.0, structured concurrency is a key concept that simplifies managing multiple asynchronous tasks. In structured concurrency, child tasks are tied to their parent tasks, ensuring that tasks are completed in a clear hierarchy, making it easier to track task execution and handle errors.
func processMultipleTasks() async {
async let task1 = performTask1()
async let task2 = performTask2()
async let task3 = performTask3()
let result1 = await task1
let result2 = await task2
let result3 = await task3
// Use the results as needed
}
This allows multiple asynchronous operations to run in parallel while maintaining structured order in your code.
2. Task Cancellation
One of the new features in Swift 6.0 is improved support for task cancellation, which is crucial for managing long-running operations like data fetching or background processing. Tasks in Swift 6.0 can be easily canceled, and developers can now check the cancellation state during task execution.
func fetchUserData() async throws -> UserData {
if Task.isCancelled {
throw CancellationError()
}
let data = try await fetchDataFromAPI()
return parseUserData(data)
}
This code ensures that tasks can be stopped gracefully if they’re no longer needed, improving performance and resource management in iOS apps.
3. Error Handling in Async Code
Swift 6.0 also continues to make error handling seamless with async functions. By using try, await, and throws in conjunction, error propagation becomes much easier to manage in asynchronous workflows.
func loadUserProfile() async throws -> UserProfile {
let profileData = try await fetchProfileData()
let profile = try parseProfile(from: profileData)
return profile
}
Task {
do {
let userProfile = try await loadUserProfile()
print("User profile loaded: \(userProfile)")
} catch {
print("Failed to load user profile: \(error)")
}
}
Best Practices for Async/Await in iOS 18 Apps
1. Avoid Overusing Task
While the Task API makes it easy to call async functions, overusing Task in your codebase can create unnecessary complexity. Aim to use structured concurrency wherever possible, and avoid creating too many detached tasks.
2. Use AsyncSequences for Streaming Data
When dealing with continuous data streams (like live updates or file downloads), AsyncSequences provide a natural way to handle asynchronous sequences of values in Swift 6.0.
for await value in myAsyncSequence {
print("Received value: \(value)")
}
AsyncSequences offer a highly efficient way to handle real-time data streams in iOS 18 apps.
3. Prioritize Task Groups for Complex Operations
Task groups enable you to group related tasks together, allowing more control over how tasks are executed and ensuring that tasks complete in a logical sequence. This is particularly useful for large iOS 18 apps that deal with multiple parallel operations.
func processTasks() async {
await withTaskGroup(of: Void.self) { group in
group.addTask { await performTask1() }
group.addTask { await performTask2() }
}
}
Task groups streamline multi-task handling, making it easier to manage concurrent workflows.
Real-World Use Cases for Async/Await in iOS 18
1. Network Operations: The most common use case for async/await in iOS apps is handling network requests, including API calls and data fetching. Swift 6.0 allows you to write simple, clean code that handles network operations asynchronously, improving both performance and code readability.
2. Database Queries: Apps that use Core Data or other databases benefit from async/await by performing database queries without blocking the main thread, ensuring smoother UI performance.
3. Background Processing: Async functions are ideal for offloading time-consuming tasks like file downloads, media processing, or data synchronization to background threads, enhancing app performance.
4. UI Updates: By combining async/await with SwiftUI, developers can ensure UI components are updated asynchronously in response to user interactions or external events, creating more responsive apps.
Conclusion: Master Async/Await for Superior iOS 18 Apps
With Swift 6.0, async/await has reached new levels of maturity, offering iOS developers a powerful and efficient way to handle asynchronous tasks. From cleaner code to better performance, mastering async/await is essential for building responsive, high-performance apps in 2024.
By following best practices and adopting Swift’s enhanced concurrency features, you can significantly improve the user experience of your iOS 18 apps while streamlining your development process.
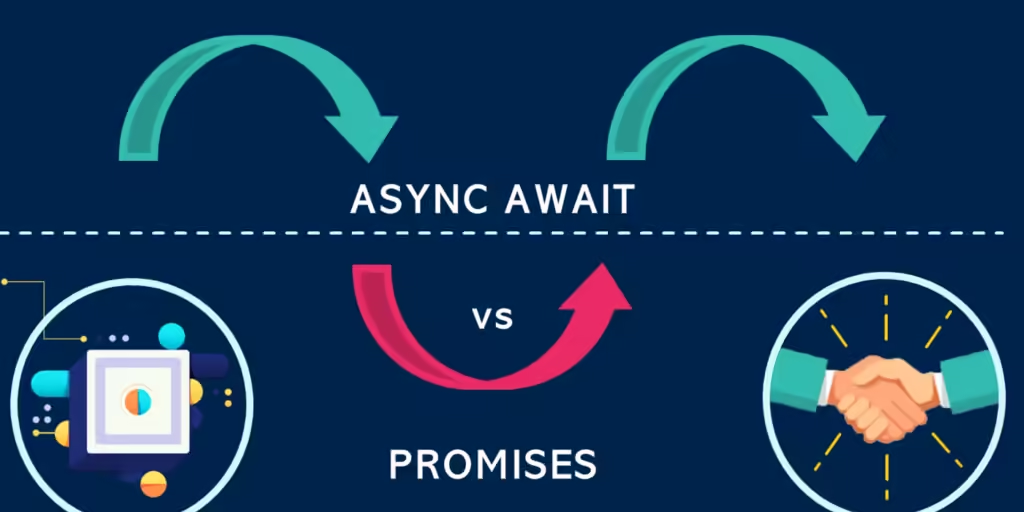
Internal Links:
• SwiftUI in 2024: Is It Ready for Large-Scale Applications?