With Jetpack Compose quickly becoming the go-to toolkit for building native UIs on Android, mastering performance optimization has become crucial for developers. As apps grow in complexity, performance considerations can make or break the user experience. In this post, we'll explore best practices for enhancing Jetpack Compose performance in 2024, ensuring your app is responsive, efficient, and enjoyable to use.
Why Focus on Jetpack Compose Performance?
Jetpack Compose simplifies UI development with a declarative approach, allowing developers to build interfaces more intuitively. However, performance issues can arise when working with larger datasets, animations, or complex layouts. Performance tuning not only improves the user experience but also leads to lower resource usage, contributing to better battery life and a faster app.
1. Minimize Recomposition
Recomposition is a core part of Jetpack Compose, where the UI updates when there is a state change. However, unnecessary recomposition can slow down the app significantly. Here are some strategies to reduce recomposition:
- Use
remember
: Use theremember
function to store values that don’t need to be recomposed, helping limit unnecessary recompositions.
val username = remember { "user123" }
- Avoid
Modifier
Changes: Avoid updatingModifier
values unless necessary, as this can trigger recomposition. Instead, make sure modifiers are only updated when essential changes occur. - Break Down Functions: Separate UI functions into smaller composables. This way, only specific parts of the UI recompose when state changes, improving performance.
2. Efficiently Manage Lists and Collections
Displaying large lists can be resource-intensive, especially when each item has complex visuals or animations. To optimize lists in Jetpack Compose:
- Lazy Lists: Use
LazyColumn
orLazyRow
instead ofColumn
orRow
for large lists. Lazy lists only render items currently visible on the screen, reducing memory usage and improving performance.
LazyColumn {
items(myDataList) { data ->
MyListItem(data)
}
}
- Stable Keys: Use stable keys for each item in a list to minimize recomposition when scrolling. This is particularly helpful when items are dynamically added or removed.
- Use Paging: For large datasets, use Paging 3 with Jetpack Compose, which loads items in small chunks rather than all at once. Paging allows for smoother scrolling and better memory usage.
3. Optimize Image Loading
Images can be one of the most significant performance drains in any app, especially when displayed in lists. Consider these best practices:
- Use Coil: Coil is a popular image-loading library optimized for Jetpack Compose. It efficiently handles caching, transformations, and memory management.
Image(
painter = rememberImagePainter(data = imageUrl),
contentDescription = null
)
- Prefer
AsyncImage
from Coil: This function from the Coil library is optimized for Jetpack Compose and minimizes recompositions when images load asynchronously. - Optimize Image Size: Ensure images are resized before loading to avoid consuming unnecessary memory, especially in lists where images are loaded repeatedly.
4. Leverage SnapshotState and Immutable Data
For efficient state management, always use SnapshotState data types like MutableState
or remember
. This ensures that only the specific components affected by a state change will recompose, avoiding entire UI re-renders.
- Immutable Data Structures: When working with complex data structures, use immutable data to avoid unintended side effects. Jetpack Compose favors immutability, which simplifies tracking changes and prevents unnecessary recomposition.
5. Limit Animations and Transitions
Animations add a dynamic feel to the UI, but too many can hinder performance. Here’s how to optimize animations:
- Use
animate*AsState
: Functions likeanimateFloatAsState
andanimateColorAsState
are optimized for Jetpack Compose and offer smooth animations with minimal impact on performance. - Limit Complex Transitions: Only use complex animations like
AnimatedVisibility
orCrossfade
when necessary. Overusing them can lead to performance issues, especially on lower-end devices. - Control Animation Scope: Apply animations selectively. For instance, animate individual composables rather than entire screens to keep transitions efficient.
6. Avoid Overuse of CompositionLocal
CompositionLocal
is powerful for passing data across composables, but overusing it can lead to memory leaks or unintended recompositions. Use CompositionLocal
sparingly, and instead, prefer dependency injection or state hoisting where possible.
- Example: Use
CompositionLocal
for static or theme-related values, like colors or fonts, and limit its usage for dynamic data that changes frequently.
7. Profile Your App
Using tools to identify performance bottlenecks is essential for effective optimization. Android Studio Profiler offers several features to analyze memory usage, rendering times, and CPU consumption.
- JankStats: In 2024, Jetpack Compose has better support for JankStats, a library that tracks and reports janky frames in an app. Identifying and fixing jank helps you keep animations and interactions smooth.
- Layout Inspector: This tool provides a detailed view of UI composition, making it easier to identify and resolve layout issues that could slow down the app.
8. Use ConstraintLayout for Complex Layouts
While Jetpack Compose is versatile, ConstraintLayout is better suited for complex layouts with many dependencies. ConstraintLayout is more efficient for positioning multiple UI elements relative to each other, reducing the need for nested layouts and improving rendering performance.
ConstraintLayout(
modifier = Modifier.fillMaxSize()
) {
val (button, text) = createRefs()
Button(
onClick = { /* TODO */ },
modifier = Modifier.constrainAs(button) {
top.linkTo(parent.top)
}
) {
Text("Click Me")
}
Text(
"Hello World",
modifier = Modifier.constrainAs(text) {
top.linkTo(button.bottom, margin = 16.dp)
}
)
}
Why Performance Optimization Matters for Jetpack Compose in 2024
As users increasingly rely on mobile apps for day-to-day tasks, performance is more critical than ever. With Jetpack Compose’s unique declarative paradigm, developers have more control but also more responsibility for managing efficient state and layouts. By following these best practices, you can ensure a smooth, fast experience for your users while avoiding common performance pitfalls.
Whether you’re building a complex app with dynamic data or a simple app with interactive animations, these techniques will help you optimize Jetpack Compose for maximum performance in 2024.
Conclusion
Jetpack Compose offers immense power and flexibility for Android UI development. By following these performance best practices, you can build apps that not only look great but also run efficiently across various devices. Keep in mind that Jetpack Compose is an evolving toolkit, with new updates and features continually emerging to make performance optimization even easier.
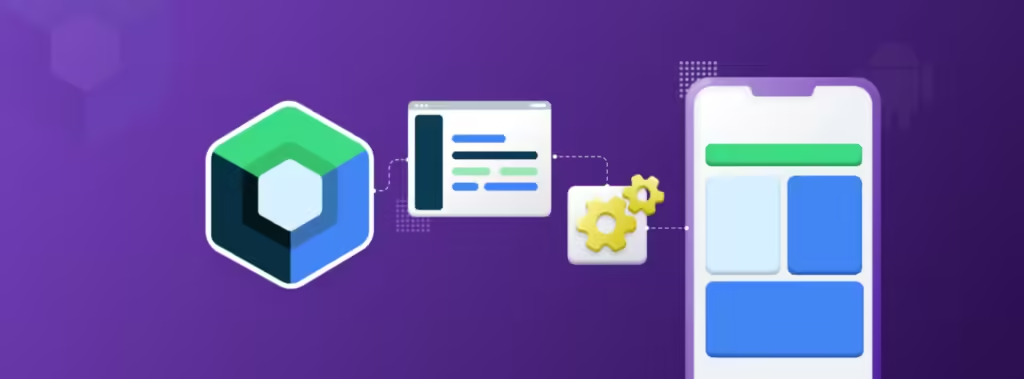
For more tips and trends in Android development, check out our Droidmate Blog.
Internal Links
For more insights into the latest android development trends, visit our Droidmate Blog and Jetpack Compose tag.
External Links
- Jetpack Compose Documentation - Official Jetpack Compose documentation by Google.
- Android Studio Profiler - Learn more about profiling tools in Android Studio.
- JankStats Library - Google’s library for tracking UI jank in Android apps.